Demo
Usage
Import the Image
component. Images are responsive by default (if you're on desktop, try resizing your browser window).
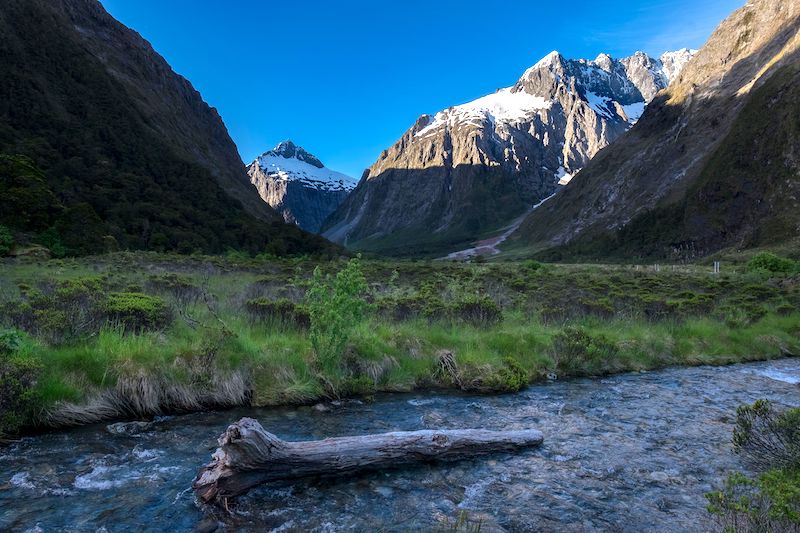
import { Image } from '@aws-amplify/ui-react';
export const DefaultImageExample = () => {
return (
<Image
src="/road-to-milford-new-zealand-800w.jpg"
alt="View from road to Milford Sound, New Zealand.
Glittering stream with old log, snowy mountain peaks
tower over a green field."
/>
);
};
Responsive Image optimization
Use sizes
and srcSet
to dynamically load different Image sizes based on screen size/resolution. For more information, see MDN responsive images article.
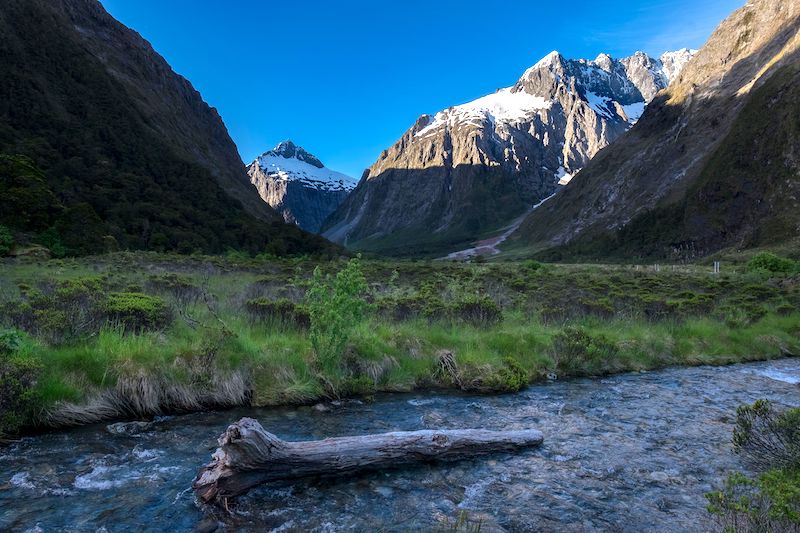
import { Image } from '@aws-amplify/ui-react';
export const ImageOptimizationExample = () => {
return (
<Image
src="/road-to-milford-new-zealand-800w.jpg"
srcSet="/road-to-milford-new-zealand-800w.jpg 800w,
/road-to-milford-new-zealand-1200w.jpg 1200w,
/road-to-milford-new-zealand-1400w.jpg 1400w"
alt="View from road to Milford Sound, New Zealand.
Glittering stream with old log, snowy mountain peaks
tower over a green field."
/>
);
};
Object fit and object position
To control how an Image fits its container, use the objectFit
and objectPosition
properties.
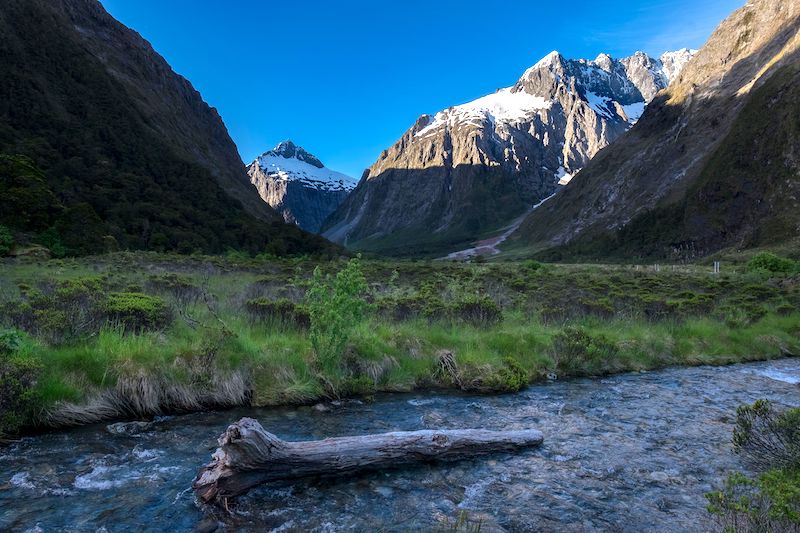
import { Image } from '@aws-amplify/ui-react';
export const ImageObjectFitAndPositionExample = () => {
return (
<Image
width="100%"
height="100%"
objectFit="cover"
objectPosition="50% 50%"
src="/road-to-milford-new-zealand-800w.jpg"
alt="Glittering stream with old log, snowy mountain peaks
tower over a green field."
/>
);
};
CSS Styling
Target classes
Class | Description |
---|---|
amplify-image | Top level element that wraps the Image primitive |
--amplify-components-image-height
--amplify-components-image-max-width
--amplify-components-image-object-fit
--amplify-components-image-object-position