Basic Usage
Did you follow the quick start instructions to set up the storage and auth services?
Next.js 13.4+ introduces App Router with the usage of Server Components. Amplify UI components are interactive and designed to work on the client side. To use them inside of Server Components you must wrap them in a Client Component with "use client"
. For more info, visit Next.js third party package documentation.
If you are using Next.js Pages Router, no changes are required to use Amplify UI components.
To use the StorageImage component, import it into your React application with the included styles.
npm install @aws-amplify/ui-react-storage aws-amplify
yarn add @aws-amplify/ui-react-storage aws-amplify
import { StorageImage } from '@aws-amplify/ui-react-storage';
import '@aws-amplify/ui-react/styles.css';
At a minimum you must include the alt
and path
props. path
is a full S3 object key and refers to the Amplify Storage path (See Download Files). It is either a string
or a callback function that accepts the current user's Cognito identityId
and returns a string
.
Public Image
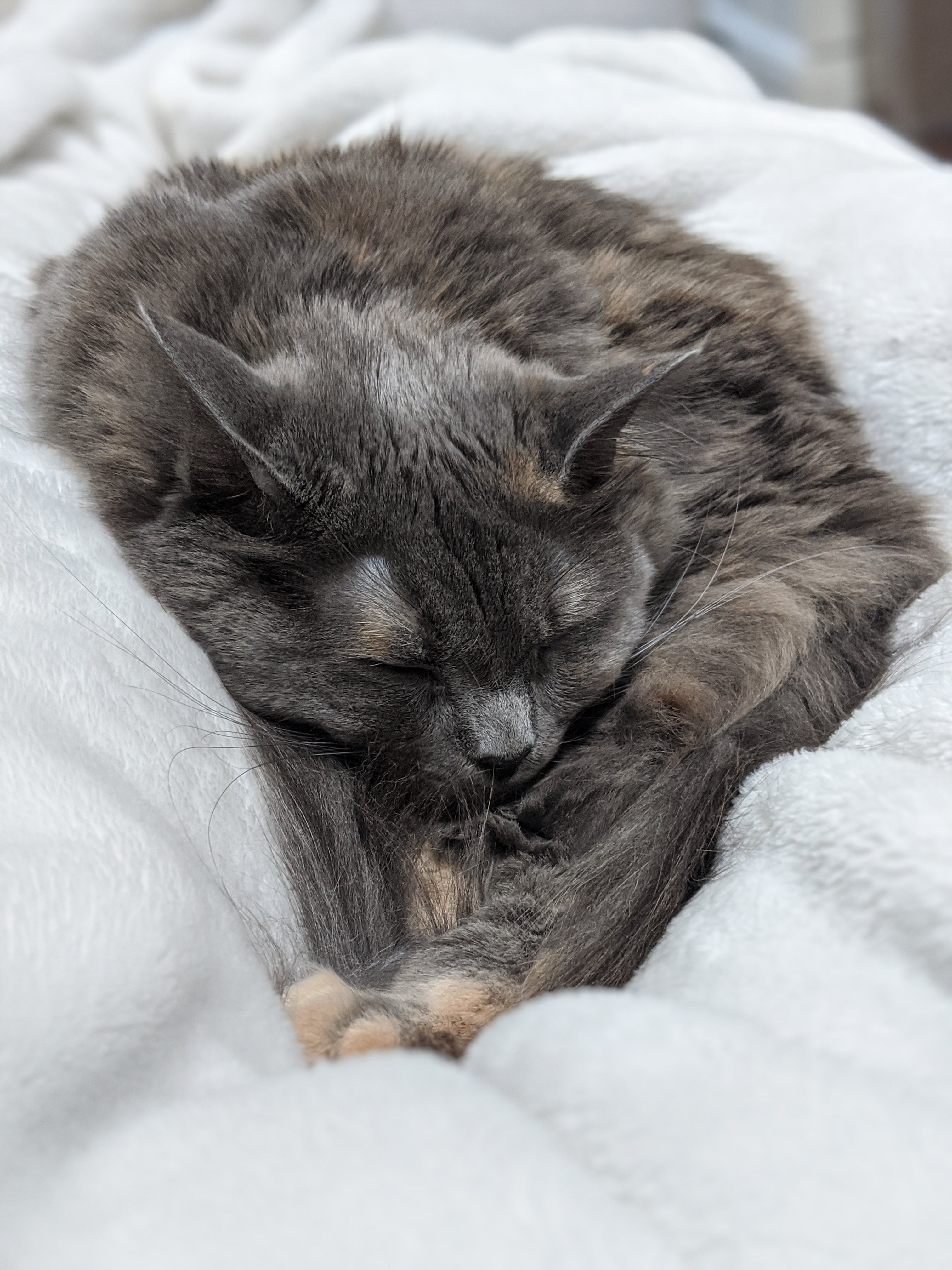
import { StorageImage } from '@aws-amplify/ui-react-storage';
export function App() {
return <StorageImage alt="sleepy-cat" path="public/cat.jpg" />;
}
Private or Protected Image
When using private or protected images, you'll need to wrap your app in the Authenticator
, allowing the StorageImage
component to infer the Cognito identityId
of the currently signed-in user. This can be done directly with the Authenticator
component or with withAuthenticator
, as shown in Add the Authenticator.
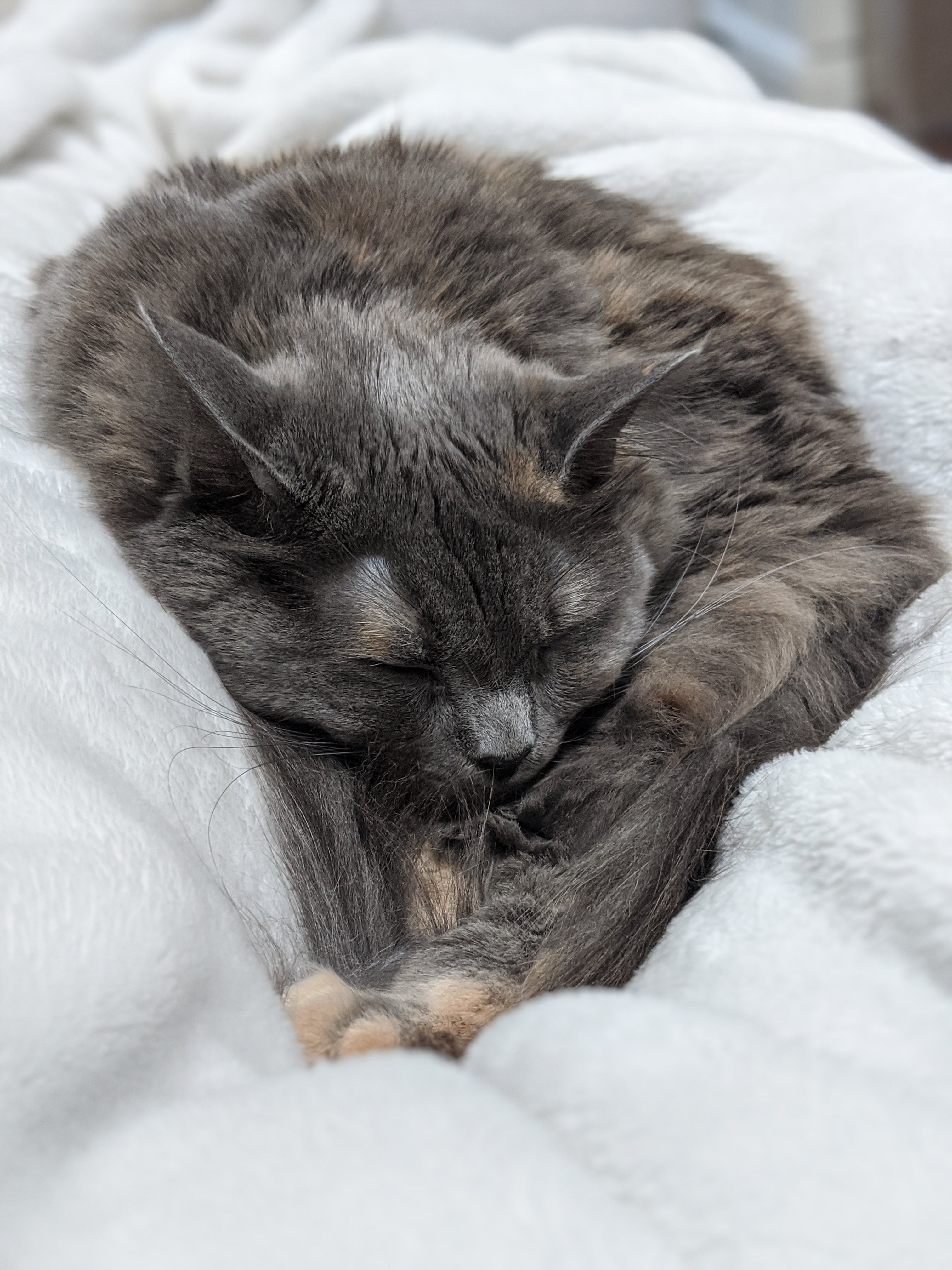
import { StorageImage } from '@aws-amplify/ui-react-storage';
export function App() {
return (
<StorageImage
alt="protected cat"
path={({ identityId }) => `protected/${identityId}/cat.jpg`}
/>
);
}
Deprecated props
Using @aws-amplify/ui-react-storage
version 3.0.18 or below?
@aws-amplify/ui-react-storage
version 3.0.18 or below?Versions 3.0.18 and earlier use imgKey
, accessLevel
, and identityId
in place of the path
prop.
<StorageImage
alt="sleepy-cat"
accessLevel="guest"
imgKey="cat.jpg"
/>
<StorageImage
alt="sleepy-cat"
accessLevel="private"
identityId={identityId}
imgKey="cat.jpg"
/>
Props
Name | Description | Type |
---|---|---|
alt | Alternative text description of the image |
|
path | The path to the image in Storage, representing a full S3 object key. See https://docs.amplify.aws/react/build-a-backend/storage/download-files/ |
|
imgKey | Deprecated, use path instead. The key of an image. See https://docs.amplify.aws/gen1/react/build-a-backend/storage/download/ |
|
accessLevel | Deprecated, use path instead. Access level for files in Storage. See https://docs.amplify.aws/gen1/react/build-a-backend/storage/configure-access/ |
|
identityId? | Deprecated, use path instead. The unique Amazon Cognito Identity ID of the image owner. |
|
fallbackSrc? | A fallback image source to be loaded when the component fails to load the image from Storage |
|
onGetUrlError? | Triggered when an error happens calling Storage.get |
|
onStorageGetError? | Deprecated, use onGetUrlError instead. Triggered when an error happens calling Storage.get |
|
validateObjectExistence? | Whether to check for the existence of a file. Defaults to true. See https://docs.amplify.aws/react/build-a-backend/storage/download-files/#more-geturl-options |
|
Error Handling
To handle the error caused by Storage.get
, you can pass a onGetUrlError
handler and optionally provide a fallbackSrc
for the component to load a fallback image.
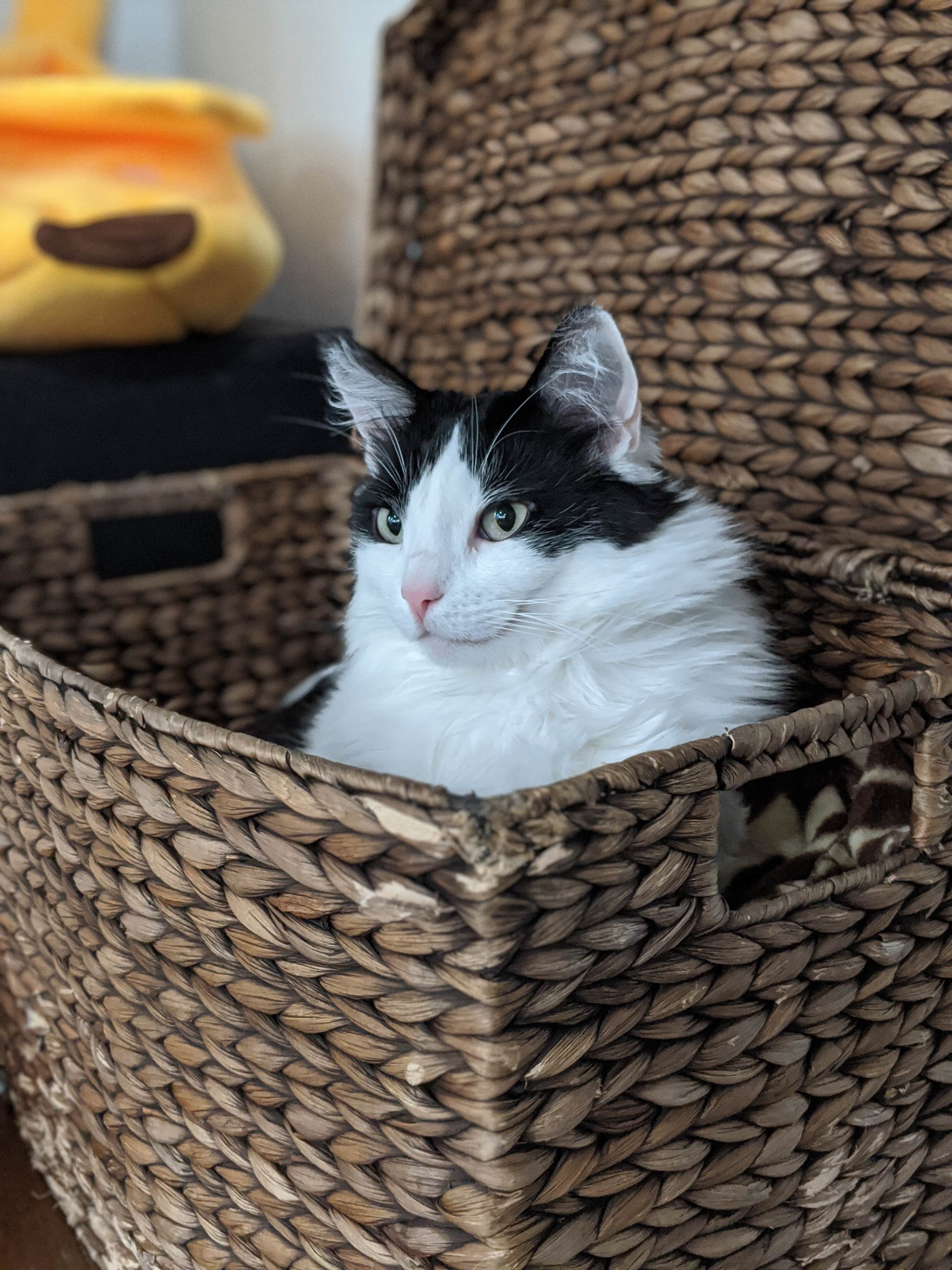
import { StorageImage } from '@aws-amplify/ui-react-storage';
export function App() {
return (
<StorageImage
alt="fallback cat"
path="guest/cat-in-basket.jpg"
fallbackSrc="/fallback_cat.jpg"
onGetUrlError={(error) => console.error(error)}
/>
);
}
Deprecated props
Using @aws-amplify/ui-react-storage
version 3.0.18 or below?
@aws-amplify/ui-react-storage
version 3.0.18 or below?Versions 3.0.18 and earlier use onStorageGetError
in place of the onGetUrlError
prop.
<StorageImage
alt="fallback cat"
accessLevel="guest"
imgKey="cat.jpg"
fallbackSrc="/fallback_cat.jpg"
onStorageGetError={(error) => console.error(error)}
/>
Customization
Target Classes
Class | Description |
---|---|
amplify-storageimage | Class applied to the img tag |